Assignment History
The program takes pride in recognizing every assignment created throughout all the cohorts and the students who were behind the creations of said assignments and the descriptions made for them, which you will find stored throughout all the years listed below:
2024



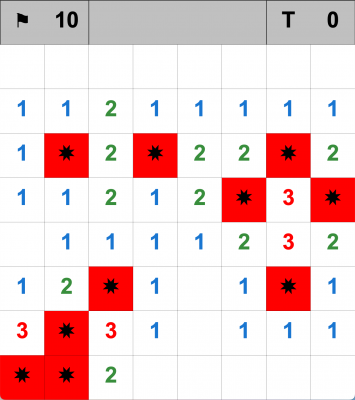
Cyber Crime Investigation – 100 course points
The purpose of this assignment is to practice using Hash Maps to analyze real-life cyber crime investigation data .
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two component:
- Early Submission (10 extra credit points) submitted through Autolab.
- Regular Submission (100 points) submitted through Autolab.
Overview
Welcome to the Cyber Crime Investigation assignment at Rutgers University!
In today’s digital age, cybercrime is an ever-growing concern, with cybercriminals becoming more sophisticated in their methods. As future cybersecurity experts, you will engage in a simulated environment where you track and analyze cybercriminals using advanced data structures. This assignment focuses on constructing and utilizing a two-layer hash table, allowing you to manage vast amounts of data efficiently and effectively.
You will be tasked with developing a `HackerDirectory` using Java, which employs hash tables to organize data about hackers and their activities. This directory will not only store detailed information about each hacker, such as their IP addresses, geographical locations, and associated cyber incidents, but it will also enable quick access and retrieval of hacker profiles and their illicit activities.
Hash tables are a crucial data structure in software development, especially useful for creating fast and efficient searchable data collections that can grow dynamically. This assignment will challenge you to implement a hash table with separate chaining to resolve collisions, which commonly occur when multiple keys hash to the same index in a table.
The primary goals of this project are:
- Implementing a Hash Table: Understand the mechanics of hashing and collision handling through separate chaining.
- Cybercriminal Tracking: Develop methods to add, search, and manage hacker data efficiently.
- Real-world Application: Apply theoretical knowledge to simulate a practical, real-world application that could be used by law enforcement agencies or private cybersecurity firms.
By the end of this assignment, you will gain hands-on experience with hash tables, enhance your understanding of complex data structures, and appreciate the importance of cybersecurity in the protection of digital assets.
This project is part of your journey in the Data Structures course, designed to deepen your understanding of algorithmic techniques and data organization, which are pivotal in developing efficient software solutions that tackle real-world problems.
By Pooja Kedia and Navya Sharma
Election Analysis – 100 course points
The purpose of this assignment is to practice using Singularly and Circularly Linked Lists, by reading and storing real US election data.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
- Coding (95 points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with their netid, and selecting “Edit your response”
Overview
Voter participation and demographics is an important aspect of any democracy, both at the local and national scales.
In this assignment, you will read real election data for the US House of Representatitves and US Senate from a Comma Separated Values (CSV) file. You will then store that data using a nested Linked List/Circularly Linked List structure. Then, we will analyze this data by year, state, and party results to gain insight into voter trends and the change in voter participation over the years.
The ElectionAnalysis class has a Linked List of YearNodes called “years”, which represent the years in which elections have taken place. Each YearNode has a Circularly Linked List of StateNodes called “states”, which represents the states which held elections in that year. Each StateNode has a Singularly Linked List of ElectionNodes, with each representing a unique election for that State in that year.
After storing this data, we can display it with the Driver to see how voter turnout and party performance have shifted in different states throughout the years.
By Collin Sullivan
Murder Mystery – 98 course points
Welcome dear debugging detectives, to a night steeped in mystery. The Mansion awaits, ready for its guests, filled with eerie rooms and items. As the sun sets, the guests enter, and the storm rages on. But unknowingly, not all will leave…
In this assignment, you will investigate a virtual murder mystery by debugging its code. The code simulates a generic board game, in which characters move through a mansion and interact with items while being pursued by a rogue murderer. Completing this assignment will help you develop your skills related to using the debugger and understanding class structures.
Check our Programming Assignments FAQ for questions about VSCode, Java, the terminal, and more.
Start your assignment early! You need time to understand the assignment code and to become comfortable with the VSCode debugger.
- See this video on how to import the project into VSCode and how to submit to Autolab.
The assignment has two components:
- Submit your auto-generated “Answers.out” file to Autolab (95 points).
- You do not need to write any code to complete this assignment.
- To generate this Answers.out, simply run the MurderMystery.java driver and answer the questions asked after the game runs.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview
It’s a dark and stormy night. The wind howls through the trees as lightning flashes across the sky. Through the fog and out of the shadows, it appears…the Mansion.
You are tasked with investigating a murder mystery, by debugging certain aspects of the simulation found within the Mansion class. – The Mansion class holds a grid of unique rooms, a cast of 6 characters (including one murderer), and a slew of items. – The rooms, players, items, player decisions, questions, and more are all randomly determined by the netID entered in the MurderMystery driver. This means that each mystery is unique, and must be solved differently.
When you run MurderMystery, you will be asked questions about the night’s events. Use the VSCode debugger to find the answers to these questions, enter them via the terminal, and then submit your Answers.out file to AutoLab.
You must use your netID when running. Your NetID is what you use to log into Rutgers systems like Canvas — don’t confuse this with your RUID (the nine-digit number). If you submit an incorrect netID or a netID that is not yours, you will receive a 0 for the assignment. Make sure you enter your netID correctly.
By Collin Sullivan
RUBreathing – 100 course points
In this assignment, you will be using Hashtable and ArrayLists to represent, manipulate, and work with Air Quality Index (AQI) values and primary pollutants for several counties for each state in the US.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
Coding (XX points) submitted through Autolab.
Reflection (3 points) submitted through a form.
Submit the reflection AFTER you have completed the coding component.
Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview
The world’s air quality is deteriorating, and you’ve been chosen as Rutgers’ leading data analyst to track air quality across various locations. Your task is to develop a robust system called “RUBreathing” that efficiently monitors and analyzes air quality data using hash tables and other data structures. In this assignment, you will implement a hash table to store air quality data for states and counties within each state, and to come up with health recommendation base on the air quality of each county! Each entry in the hash table will be indexed by a state/county code, allowing for efficient retrieval and updating of data.
By Srimathi Vadivel, and Anna Lu
RUMaps – 100 course points
The purpose of this assignment is to practice your understanding of Directed Graphs and Adjacency Lists, and work with streets and intersections in the Rutgers-NB Community.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
Coding (XX points) submitted through Autolab.
Reflection (3 points) submitted through a form.
Submit the reflection AFTER you have completed the coding component.
Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview
You will be creating mini google maps for Rutgers’ community. Of course you will not be required to do anything with visuals, this part will be provided in the zip file. In this assignment you will be given an input file that contains information about the streets within the New Brunswick and Piscataway area. With these information you will be able to initialize the street, blocks, intersections and be able to do the search methods such as DFS, BFS, and Dijkstra’s (provided with pseudocode) afterwards.
By Vian Miranda, and Anna Lu
Character Counter – 100 course points
The ASCII (American Standard Code for Information Interchange) has a decimal value/representation for encoding 128 different letters, punctuation and other characters. In the age where millions of bytes of data are stored and sent across networks with a single click, it’s important to optimize the memory and time of the computers that carry out these tasks.
Huffman Coding is a method of data compression that counts the occurences of each character and maps them to a certain bit string based on their frequency for optimized space.
Video about Huffman Coding Method
More reading about Huffman
In this assignment, you will be implementing your own program that will keep track of the number of occurances of these 128 characters and the percentage of the entire text file that each character represents. This is the first part of the Huffman Coding algorithm.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
Given an input file, students are then required to create a program using two parallel arrays that returns the total number of times and total percentage that each character appeared in the input file and print to the output file.
You can see that at index 65 of the int array that tracks, the value is 9 because 'A'
appears 9 times in the input file and the ascii value of 'A'
is 65.
By Mary Buist, and Anna Lu
Elevator – ## course points
The purpose of this assignment is to familiarize you with using arrays.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
By Vidushi Jindal, and Pooja Kedia
Long ago, some advanced civilizations such as the Egyptians modeled their architecture to be of pyramid form: 3-Dimensional polyhedra with some polygon base and triangle-shaped flat sides meeting to a point at the top. For the sake of this assignment, we will be considering 2-D pyramids, which take the form of triangles. You will be tasked with understanding and implementing 2D array(s) to create a 2-Dimensional pyramid of your own.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Oh no! During Professor Centeno’s 2D Arrays lecture, a mysterious portal opened up right into the projected picture of the Egyptian pyramids, sucking all the CS111 students into another time dimension. It looks like the students are trapped in 2580 B.C. After some conversing (let’s assume due to advanced language translation technology that students happened to bring), they discover that the Egyptians need assistance in building their pyramids. Since Professor Centeno did not time travel with the CS111 students, it’s now up to them to help these Egyptians out.
The students need to code a program-taking in two tentative parameters from the terminal representing 1) the overall size of their figure and 2) the number of bricks provided to build a pyramid.
By Kushi Sharma, and Ayla Muminovic
Fingerprints – ## course points
This assignment’s purpose is to introduce you to OOP in Java through biometric data analysis as a means of security authentication.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
- Coding (XX points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview
This assignment uses Java OOP to simulate onboarding a set of employees into a company. You will read from a csv file and create People objects to add to the company. Using a driver, you will add and remove employees, login and logout employees and run reports.
By Sumedh Sinha, and Mary Buist
Floor Is Lava – ## course points
The purpose of this assignment is to familiarize you with loops and conditions in java, as well as to practice writing methods and understanding sequences.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
In this assignment, you will write a program that simulates a sequence of safe spaces (stones), and dangerous spaces (lava) based on a given range of numbers. Your task is to print all safe spaces (even numbers) in ascending order first, then all remaining safe spaces in decreasing order after a switch where stones become odd and lava biomes even
By Shane Haughton, and Maaz Mansuri
Fruit Costs – ## course points
This assignment is designed to help you practice working with arrays and conditional statements. The purpose of this assignment is to write a program that reads in a list of fruits and their costs, then prints out the names and costs of the two lowest-cost fruits, and their total cost.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
Picture this: it’s a sunny day, and you’re craving a refreshing fruit smoothie to start off your day. As a college student, you’re mindful of your budget, so you want to find the two cheapest fruits to make your smoothie without overspending. Using real fruit prices (https://catalog.data.gov/dataset/fruit-and-vegetable-prices), you’ll be creating a program to assist you in making sure you get your fruits without breaking the bank.
The program will process a list of fruits and their corresponding costs, identify the two fruits with the lowest costs, and calculate their combined total. This way, you can enjoy your smoothie without worrying about the price. As you work on this task, you’ll get to practice using arrays, loops, and basic arithmetic operations in Java, all while ensuring you make the most out of every dollar you spend on fruits.
By Srimathi Vadivel, and Sarah Benedicto
Headline Search – ## course points
The purpose of this assignment is to give CS111 students exposure into Object Oriented Programming thinking and logic.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
- Coding (100 points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview
The core intent of this assignment is to give students exposure to real world data analysis using Java. All the CSV files provided contain lists of headlines that were released in Huffington Post from the years of 2012 to 2022. Using this data students will interpret and and analyze these headlines and draw “newsbreaking” conclusions (haha) about the headlines provided. Students will learn about searching for keywords, phrases, and just get a general understanding of how search engines work.
By Tanvi Yamarthy, and Julia Dymnicki
Quadratic Koch – ## course points
The purpose of this assignment is to practice recursion through writing a program that plots the Quadratic Koch Curve, a recursive design that is a variation of the commonly known Koch Snowflake. To learn more about the Koch Snowflake and other recursive designs, please visit https://en.wikipedia.org/wiki/Koch_snowflake.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
The Quadratic Koch Curve is an example of a fractal pattern like the H-tree pattern from Section 2.3 of the textbook.
The original Koch Snowflake was created by the Swedish mathematician Helge von Koch, who described the pattern in 1904. Your main task is to write a recursive function koch()
that plots a Quadratic Koch Curve of order n to standard drawing.
By Jeremy Hui
Matryoshka Doll – ## course points
The purpose of this assignment is to apply the concept of recursion by recursively generating a series of dolls to resemble the popular Russian nesting doll set, Matryoshka Dolls, which are a series of wooden dolls that are of decreasing size nested within one another.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
Matryoshka Dolls are also known as nesting dolls that are of Russian origin. They consist of a set of dolls where each doll is progressively smaller than the one before such that they can be placed within one another.
As depicted here, in this assignment we will model the recurring design of the dolls as they decrease in size from left to right.
By Pooja Kedia
Hansel And Gretel – ## course points
In this assignment, you will model a breadcrumb trail in order to write a method that can find the exit of a maze. You will traverse a 2D array using recursion, and use 2D array manipulation to generate paths of return.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
Overview
In the fairy tale Hansel and Gretel, the two main characters find themselves exploring a forest. To find their way home and ensure they don’t get lost, they mark their return path with breadcrumbs. In this assignment, you are tasked with helping Hansel and Gretel find their way out of the forest maze using recursion.
The method that you will implement is a basic form of a depth first search, a method used for searching certain data structures. In our case, it can be used to search a 2D array, or maze, for an exit. To learn more about what a depth first search looks like on a maze, you can watch this video (0:24- 1:28).
The maze, or forest, will be passed in as a 2D array of booleans. Spaces with the value true will be pathways that Hansel and Gretel can travel, and spaces with the value false will represent walls, or boundaries that the characters cannot cross. An example of one maze or forest is pictured below
It is important to remember that the START will always be row 0, column 0, and the FINISH will always be the last space (maze.length, maze[row].length). You will be tasked to traverse this 2D array using recursion, placing breadcrumbs on each new space that you visit. Placing these breadcrumbs will therefore allow you to backtrack when you reach a dead end, a process you can repeat until you find the maze’s finish.
By Maaz Mansuri, and Shane Haughton
In this assignment, you will use OOP and 2D arrays to create game boards and implement methods according to the rules of the logic puzzle video game, Minesweeper.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the files into VS Code and how to submit into Autolab.
The assignment has two components:
Coding (XX points) submitted through Autolab.
Reflection (3 points) submitted through a form.
Submit the reflection AFTER you have completed the coding component.
Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response.”
Minesweeper is a logic puzzle video game featuring a grid of clickable squares with hidden mines scattered throughout. The objective of the game is to open all of the squares in the grid without detonating any mines. Each square displays a number clue that reflects the number of mines neighboring each square. Players can use these number clues for help navigating the minefield — flagging squares they suspect contain mines and opening squares they assume are safe. Be careful not to click on a mine because if you do . . . it is game over!
For this assignment, you are tasked with developing a program that accomplishes the same objectives as and adheres to the rules of Minesweeper.
To accomplish this, you will work with a 2D array of Square objects, each representing an individual game piece and storing information like the square number and the square state.
By Jeremy Hui and Jessica de Brito
Overview:
How would you share a secret message with someone that you’ve never met in a room full of eavesdroppers?
The purpose of this assignment is to teach students about public-key cryptography by simulating secure communication between two computer systems, referred to as Alice and Bob. In this simulation, Alice and Bob will exchange encrypted messages using public-key cryptography techniques to ensure that their communication remains confidential, even in the presence of a potential eavesdropper. This assignment will demonstrate how public-key cryptography can be used to protect sensitive information and ensure secure communication over an insecure channel.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
- Coding (XX points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
By Jeremy Hui and Tiara Clyde
RU Racing – ## course points
Welcome to RURacing! You’re familiar with runtimes and Big O notation, right? Well, we’re bringing that to the racetrack! We’re going to create a raceway that will have racers moving through it at different runtimes. We will start with a simple raceway and move on to more complex ones. Each racer will move a certain distance through the raceway based on their given runtime (i.e., O(1), O(n), …, etc.). Do not let racers run into the grass!
Ready? Set? Go!
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line, and how to submit your assignments.
We provide this ZIP FILE containing RURacing.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it to Autolab.
Overview:
This assignment will teach students about runtime and the importance of Big O notation by tasking them with programming racers that move at different “speeds.” Each speed will correspond to the successful completion of one lap on a given raceway.
For example, a racer with an O(1) runtime will complete a full lap in a single step, demonstrating constant time complexity. In contrast, a racer with an O(n) runtime will take n steps to complete the lap, illustrating linear time complexity. Through this exercise, students will visually observe how different algorithmic efficiencies impact performance and understand the practical implications of various runtimes in algorithm design.
Students will be given different test tracks stored within “track” files as a set of points stored in plain text to construct raceways. These points will be drawn upon a 2D matrix (character array) where we will determine if racers are moving at the correct pace and direction.
The construction step of the 2D array will be delegated to a separate method, createRaceway(), which students are responsible for completing.
NOTE – If you require a refresher on 2D arrays, you can refer to your lecture slides or notes. Princeton also offers an overview based on your book.
The 2D array will be interpreted in the following manner:
- Points on the array will be represented by x and y coordinates.
- The x-coordinate will be the column and the y-coordinate will be the row.
- Points will be stored in an (x, y) format.
- The top-left corner of the map will be (0, 0). The bottom-right corner will be (size – 1, size – 1).
An image of a 2D array with an embedded raceway and example coordinates is provided below:
From now on we will refer to this 2D matrix/array as a “map,” which includes all text characters. All characters that represent this map have been provided as constants (GRASS & ROAD). The ‘#’ character represents the road racers will run on, and the ‘.’ character represents grass (what racers should not run on).
By Elian Deogracia-Brito, Tanvi Yamarthy, and Tiara Clyde
In this assignment, you will use OOP and ArrayLists to represent, manipulate, and work with solar panels and parking lot projects.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
Coding (XX points) submitted through Autolab.
Reflection (3 points) submitted through a form.
Submit the reflection AFTER you have completed the coding component.
Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
Overview:
Rutgers has hired you as a solar panel consultant – congrats! You’re in charge of setting up solar panels on top of parking lots – right now, Rutgers has a large number of solar panels to generate electricity and reduce carbon emissions, but you’ll need to work to lay out more panels given a set of maps.
More specifically, you will work with the following:
A street map containing parking lots (always labeled “P#” where # is a number) and other road features that are solely meant for decorations. Don’t place a solar panel in the middle of a road!
An ArrayList of parking lot objects, containing info like budget, parking lot, max panels, and capacity.
An array of Panel objects that contain individual solar panels at particular positions. This array has the same size as the street map, and a certain position [i][j] refers to THE SAME position in the street map.
2023




Braille Translator – 100 course points
Overview
Braille is a tactile writing system used by people with visual impairments. It was developed by Louis Braille in the early 19th century and consists of patterns of raised dots arranged in cells. Each cell contains up to six dots, representing letters, numbers, and punctuation. People read Braille by feeling these dots with their fingertips, allowing them to access written information through touch.
In this assignment, you will use Binary Search Trees to build a symbol table of Braille encodings (a sequence of six digits, each either 0 or 1). 0 means that the dot is lowered; 1 means that the dot is raised. We can represent this with node directions – intermediate nodes have null symbols. 0 moves a pointer to the left while 1 moves it to the right until you reach a leaf node. The diagram below shows tracing paths for two letters.
Note that this assignment will only cover translating lowercase, alphabetical characters. Braille uses abbreviations to represent different words; for simplicity we have omitted those.
By Kal Pandit and Seth Kelley
Climate and Economic Justice – Exploring Community Data – 98 course points
Overview
Welcome to the Climate and Economic Justice assignment! In this task, you’ll be working with community data from various regions to explore the intersection of climate and economic justice. The provided data includes information about racial demographics, pollution levels, flood risks, poverty rates, and more. Your goal is to process this data using linked lists and analyze the social and environmental aspects of different communities.
In this assignment, you’ll implement methods in the ClimateEconJustice.java class to create a 3-layered linked list structure consisting of states, counties, and communities. Each layer is interconnected, allowing you to navigate through the data hierarchy. Your methods will carry out operations on this linked list structure to gain insights from the community data.
This assignment has two components:
- Coding (95 points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with your netid, and selecting “Edit your response”
By Navya Sharma
Forensic Analysis – ## course points
The purpose of this assignment is to practice using Binary Search Trees to analyze DNA.
Overview
You’ve been tasked with identifying who a DNA sequence may belong to based on an unknown person’s DNA sequence and a DNA database you have. A person’s DNA has pairs of chromosomes; we will focus on a single pair of the same chromosome to identify people whose samples we want to investigate further.
DNA is a molecule found in living organisms that carries our genetic information. It is made up of nucleotides, which are organic building blocks that consist of a base, sugar, and phosphate. Nucleotides contain one of four different bases – adenine (A), thymine (T), cytosine (C), and guanine (G). We will focus on combinations of these four bases in this assignment.
99.9% of our DNA is the same as other humans. Our goal is to focus on the 0.1% of DNA that varies between humans – these are sections of “noncoding DNA” that are in between genes and don’t provide instructions for making proteins. Short Tandem Repeats, or STRs, are in noncoding DNA and are short sets of bases that are repeated across a sequence.
In this assignment, you will work with simple DNA sequences with 2-5 STRs. We will identify the number of times a set of STRs is repeated within a sequence to find out who a pair of unknown DNA sequences may belong to in a DNA database.
Why BSTs? In a past semester of CS111, students had a DNA assignment that used an array of objects to store a DNA sequence and find hereditary relationships. When we have a large number of items, we can add/remove a single item more efficiently using a BST in O(log n) time than an array, which takes O(n) time. Do you understand why this is?
- This assignment differs significantly from the CS111 assignment: it focuses on BST operations to access and flag profiles rather than string and object operations to calculate STRs within profiles. We provide you with the STRs.
- Here’s a diagram that shows how we are using nodes to store (name, profile) pairs.
By Kal Pandit
Food Web Graphs – 100 course points
This assignment aims to create food web graphs
Overview:
Ecosystem ecology aims to understand how energy and materials enters, interacts with, moves through, and eventually exits an ecosystem. The core concept in the study of how energy moves through the ecosystem is the Consumer-resource interaction.
The concept of where energy flows in an ecosystem is studied by understanding the hierarchy of consumption organizing the denizens of the ecosystem into trophic levels.
Ecologists aim to study the relationships between organisms and their environment. An important relationship that ecologists study is the feeding relationships between species and how energy flows through the system. The dynamics of consumer-producer relationships and energy in an environment flow forms a complex network of relationships. In order to tame this complexity ecologists model the relationships as a food-web.
A food web models energy flow as consumers and producers. Where a producer and consumer are connected if the consumer species eat the producer species in order to obtain energy. The direction of energy flow is from producer to consumer.
This type of relationship can be conveniently modeled as a Digraph, opening up a host of algorithms to assist with the structural analysis of energy flow through ecological systems.
Food webs can be used to model and study the impact and spread of runoff and pollution in an ecosystem.
By Joseph Granick, and Sreshta Myana
View full description and materials here: http://nifty.stanford.edu/2024/ravi-centeno-infinity-war/
Infinity War – 100 course points
The purpose of this assignment is to practice your understanding of graphs and the adjacency matrix graph storage.
This assignment WILL TAKE A SUBSTANTIAL AMOUNT OF TIME TO COMPLETE.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Overview
We do not expect that you have seen the movie in order to complete this assignment. This description will give you enough background information for you to understand how to complete each task.
In the MCU (Marvel Cinematic Universe), right after the Big Bang occurred there were 6 singularities contained within Infinity Stones that were spread across the infant universe.
Backstory of Thanos
Before the events of Avengers: Infinity War, a titan named Thanos witnessed the death of his home planet due to overpopulation. Reeling from his experience, Thanos made it his life mission to exterminate half of all life in the universe to delay overpopulation on other worlds like his. Thanos determined that using the 6 Infinity Stones which he could hold in his Infinity Gauntlet, a simple snap of his fingers would painlessly eliminate 50% of all life, and he can finally rest in peace victorious.
The Infinity War
If you are interested in the film’s story the following videos will contextualize it for you.
TRAILER: Marvel Studios’ Avengers: Infinity War Official Trailer
The film begins with Thanos destroying Thor’s homeworld (Asgard) after acquiring the Power and Space Stones.
- Avengers: Infinity War (2018) – “Attack On The Statesman” | Movie Clip
- Thanos Kills Loki Loki Death Scene Avengers Infinity War 2018Lifeline HD
A defeated Hulk is sent to NYC to warn Dr. Strange and Iron Man about the impending arrival of Thanos and his army (the Black Order) to retrieve the Time Stone.
His ship arrives soon and a battle ensues as Iron Man, Spiderman, and Dr. Strange battles Ebony Maw and Cull Obsidian (two of Thanos’ “children”).
After Cull Obsidian dies, Dr. Strange is captured onto Maw’s ship, and Iron Man and Spiderman latch onto it as it departs Earth. Iron Man blows a hole in the ship and Maw is shot into space.
Meanwhile, the Guardians of the Galaxy (hereby referred to as “Guardians”) rescue Thor and the group splits, where Thor, Rocket, and Groot (a tree-human hybrid) go to Nidavellir to forge Thor’s Stormbreaker, a weapon capable of destroying Thanos.
By Yashas Ravi
Great Pacific Garbage Patch – ## course points
THIS ASSIGNMENT IS LONGER THAN PREVIOUS CS ASSIGNMENTS. START EARLY; DO NOT WAIT UNTIL THE LAST MINUTE TO SUBMIT!
Overview
The Great Pacific Garbage Patch is a massive area of the Pacific Ocean that is contaminated with waste and debris. It is located between Hawaii and California and is estimated to be twice the size of Texas. The patch is formed due to the swirling currents in the ocean, which bring together the waste materials and trap them in a particular area. Plastic is one of the most common types of waste – it does not biodegrade but rather breaks down in smaller pieces. This poses a threat to marine life as some animals mistake plastics and other waste for food and can even become entangled in nets. The accumulation of waste in the Great Pacific Garbage Patch even poses a threat to the food chain – plastics can absorb and release pollutants and chemicals, and algae and plankton can become trapped.
In this assignment, you will use 2D arrays, ArrayLists, and weighted quick-union to simulate debris flow in the Great Pacific Garbage Patch. You will create a representation of an ocean and use array and weighted quick-union operations to simulate current flow, process garbage breakdown, and identify the number of debris islands in a section of the garbage patch.
By Kal Pandit and Seth Kelley
Music Playlist – 100 course points
In this assignment, you will create an implementation of a music streaming application. You will practice circular linked list manipulation, referencing objects, reading from CSV files, and the object-oriented programming (OOP) paradigm.
Overview
Apps such as Spotify, Apple Music, and Pandora are applications known as music streaming services. They allow users to stream digitally copyrighted music or on-demand music by joining a subscription. This is done by having songs that are being played point to a reference to a song on the streaming service’s database, which allows for better space efficiency than copying the song into the user’s device. Each song also holds metadata (or “data about data”) for each song, as having proper accreditation is necessary to ensure artists and songwriters receive proper compensation. Read more about what metadata is and why it matters here.
For this assignment, you will assume that the database is centralized in the music folder and that each of the music objects holds a “link” to each song a user may add to their playlist. Therefore, playlists (which are implemented using circular linked lists) can be flexibly created by having a reference to the song object rather than storing the song itself. Once the implementation of the music library is complete, you will even be able to play the playlists you create!
By Jeremi Hui and Vian Miranda
Rising Tides – ## course points
In this assignment, you will see the effects of rising sea levels on various major cities around the world using 2-D arrays. You will practice array manipulation, referencing objects, the Weighted UnionFind algorithm, and the object-oriented programming (OOP) paradigm.
Overview
Rising sea levels have become an increasingly urgent issue due to climate change. Your goal is to create an effective visualizer of the impacts these increases in sea levels will have on various terrains.
By Vian Miranda
RU Hungry – 100 course points
Overview
You are going to be running RUHungry for a day by creating a menu, a stockroom (with all the ingredients to make your menu items), an overall summary of all the transactions of the day(including taking customer orders, donations for the food pantry, and restock orders for the stockroom), and finally, seating guests at tables dependent on how many guests there are and the size and availability of tables.
As you may or may not know, the Rutgers Pantry is an integral part of Rutgers student life especially for those students who face food insecurity. But not only does the pantry have food but because the pantry is a partnership with the Off Campus Living and Community the pantry also provides contacts for campus housing information and commuter information. Since March 2020, there have been around 3,000 student visits to the pantry. Remember food insecurity doesn’t mean starving, it refers to the state of an inadequate food supply or unreliable food source.
And because of this huge impact on the Rutgers NB student population, donations are always welcome and encouraged. However there are many other ways to help the pantry like volunteering as an ambassador through social media promotion or just spreading awareness to hundreds of students… like this assignment is doing!
Your assignment is to run RUHungry for the day (*disclaimer* this assignment is NOT affiliated with RUHungry in any capacity however RUHungry is located at the Yard for anyone who does want a fat sandwich or a milkshake) by seating guests, taking orders, donation requests and restocking the pantry. As all good businesses should do, you will be keeping track of which parties are sat and when, as well as printing an end of day receipt for every single transaction regardless of its success.
A visual of the provided files and methods are included below as well as a screenshot for each test case method within the driver. There are quite a lot of classes and objects which we will provide. DO NOT EDIT any other files except the RUHungry file (which you will submit). Those combined with written instructions will help you complete the assignment.
Enjoy the data structures pick up lines sprinkled throughout the assignment but please don’t use any of them in real life (since their success rate is astronomically low). Good luck!
*there are a lot of people in the Computer Science department but I like to think I’m an exception; if I throw myself at you, will you catch me?*
By: Kushi Sharma and Mary Buist
Spider-Verse – 98 course points
The purpose of this assignment is to practice your understanding of Undirected Graphs and Adjacency Lists.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
- See this video on how to import the project into VSCode and how to submit into Autolab.
The assignment has two components:
- Coding (95 points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with their netid, and selecting “Edit your response”
Overview
Welcome to the Spider-Verse!
In the Spider-Verse there are many different Dimensions and just as many Spider-People. As you would’ve guessed, with great power comes great responsibility and these Spiders are tasked with protecting their Dimension from any threat that may hurt anyone.
To help visualize the Spider-Verse, we can make a graph of all the different Dimensions, with some holding any number of Spiders and any number of anomalies. Anomalies are people (mainly villains) who have been sent to a different Dimension as the result of the mob boss named Kingpin running dangerous Particle Collider experiments in Dimension 1610.
Dimension 1610 is home to Miles Morales, one of the many Spider-men, and is also the source of the anomalies. We need to set them straight along with finding out some useful information about graphs along the way. In each method you will learn some backstory for the method, which will be implemented by you.
You DO NOT need to be familiar with Spider-Man or the Marvel Cinematic Universe in order to complete this assignment.
By Seth Kelley
Assignment DNA Analysis – ## course points
In this assignment you will use Object-Oriented Programming to look at DNA and analyze it to find out useful information.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing DNA.java. Update and submit the file on Autolab.
Overview
DNA is the carrier of genetic information that all living things are made of. Any individual’s DNA can be observed by looking at any of the chromosomes that individual has; where the chromosome is the structure that holds the DNA . We can use this information about a person’s DNA to tell us useful things about said living things, such as finding out who it belongs to or where it came from (such as relatives).
How can this be accomplished though? DNA is composed of genes (which all humans share), and “junk” DNA (sections of non-coding DNA in between the genes that are highly variable between individuals). By looking at these sections of “junk DNA” we can accomplish our goal.
What exactly are we looking for in this junk DNA? Inside of these sections of the DNA, since there is a high variance of what’s in there, we can uniquely identify individuals based on what’s in there by looking at STRs (Short Tandem Repeats).
What are STRs and how can I use them to tell me what I want to know? STRs are Short Tandem Repeats, which are short sequences of DNA that repeat back-to-back in every person a variable number of times.
AGAT and ACGT are examples of short DNA sequences, which we can use as STRs.
Let’s take a DNA sequence which looks like this:
AGATAGATAGATACGTACGT
Here, you see that the STR “AGAT” is repeated three times followed by another STR “ACGT” repeated twice (this is just one example of many possibilities). The more STRs we use to analyze a DNA sequence the more refined our list will be to find out the information (such as who it belongs to) we desire. When looking at these STRs in a DNA sequence we want to look at the longest number of times that given STR is repeated as well to properly find the highest variance of the STR.
The FBI uses 20 different STRs when processing DNA for their Combined DNA Index System (CODIS) database. For simplicity, we will be using 2 to 4 STRs that are a few nucleotides long. These nucleotides are the building blocks of DNA, called Adenine, Cytosine, Guanine, or Thymine.
The goal of this assignment is to carry out a DNA profiling method called STR analysis, where we look at these STRs to accomplish the goals of finding out who it belongs to as well as finding relatives (parents in our case) of a sequence.
By Seth Kelley and Aastha Gandhi
ISBN Calculator – ## course points
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing StopAndFrisk.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it into Autolab.
Overview
The International Standard Book Number, or ISBN, is a number that uniquely identifies a book. Publishers can buy or receive ISBNs from the International ISBN Agency. There are two types of ISBNs – ISBN-13 and ISBN-10, which are 10- and 13-digits long, respectively.
ISBN-10 has a pattern, where the last digit can be found using the first 9. Additionally, ISBN-10 can also be used to calculate its ISBN-13 equivalent (and vice versa).
By Aastha Gandhi
Stop And Frisk – 100 course points
In this assignment, you will analyze real CSV data from the New York Police Department from 4 prominent years: 2013, 2014, 2021, and 2022. Each of these files consists of approximately 10,000 Stop and Frisk cases that occurred in that year. You will practice reading in data from CSV files, using arrays and ArrayLists, referencing objects, and the object-oriented programming (OOP) paradigm.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing StopAndFrisk.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it into Autolab.
Overview
The goal of this assignment is to allow you to draw conclusions about social bias using real data from the New York Police Department. In 1964, the New York State passed a policing law called “Stop and Frisk” which enables officers to stop and “frisk” (briefly search) a person that they deem to be suspicious. A person who is stopped has not necessarily committed a crime; people are presumed innocent until proven guilty by the courts. However, data has shown that there exists significant discrimination, including racial and gender bias, regarding which individuals are more likely to be stopped.
Feel free to read more about the history of the Stop and Frisk Policy in New York to get a better understanding of social bias’ it indicates: https://www.nyclu.org/en/closer-look-stop-and-frisk-nyc
This assignment will allow you to deeply explore four years in New York’s Stop and Frisk history which allows you to see the difference (if any) in Stop and Frisk logistics from 2013 and 2014 to more recent years (2021 and 2022). These years were specifically chosen to analyze during and after the policy was put into place. You will implement a total of 6 methods to analyze and broaden your understanding of this topic.
By Tanvi Yamarthy and Vidushi Jindal
Seam Carving – Content-Aware Image Resizing (100 points)
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing SeamCarver.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it into Autolab.
Overview
Welcome to the “Seam Carving” assignment! In this project, you’ll be diving into the fascinating world of content-aware image resizing using the “seam carving” technique. Seam carving lets us resize images in a way that preserves important features and maintains the aspect ratio, which is especially handy for retaining the integrity of images.
In this assignment, you will be working extensively with the SeamCarver.java class. Your goal is to fill in various methods that facilitate image manipulation using the seam carving technique. Seam carving involves finding and removing paths of pixels (seams) from an image based on their energy. The energy value of each pixel is indicative of its significance in the image.
For more information, visit the Princeton website which further details this process.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing SeamCarver.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it into Autolab.
By Navya Sharma, and Seth Kelley
RU Doggy Daycare – ## course points
In this assignment, you’ll step into the world of canine care by crafting a Doggy Daycare Management System. You’ll get to work with 2D arrays, object-oriented programming (OOP), file reading, and methods. Through this project, you’ll gain practical insights into coding logic, data representation, and problem-solving while keeping our furry companions in good hands.
Overview
Welcome to the “A Weekend at RU Doggy Day Care” assignment! In this task, you’ll delve into the world of object-oriented programming (OOP) as you simulate the operations of a doggy day care center. This assignment will challenge your OOP skills as you create and manipulate Dog objects, manage dog check-ins and check-outs, handle premium memberships, calculate costs, and track daily revenues. Through this project, you’ll gain practical insights into coding logic, data representation, and problem-solving while keeping our furry companions in good hands.
Imagine you are the manager of the RU Doggy Day Care, a bustling center that takes care of adorable dogs during the weekend. Your task is to develop a program that assists with the daily operations of the day care. You will be working primarily with the DoggyDayCare.java class, which is the heart of this simulation.
This program involves managing dog check-in and check-out times, keeping track of premium memberships, calculating the cost of each dog’s stay, and computing daily revenue. Additionally, you’ll need to sort dogs into different batches based on their check-in times and days of the weekend.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
We provide this ZIP FILE containing RUDoggyDaycare.java. Update and submit the file on Autolab.
See this video on how to import the project into VSCode and how to submit it into Autolab.
Skills You’ll Utilize:
- Object-Oriented Programming (OOP): You’ll create and manipulate objects of the Dog class, organize them into arrays, and use their attributes to perform various tasks.
- Data Processing: You’ll read data from a CSV file (DoggyData.csv), parse it, and populate an array of Dog objects.
- Sorting and Categorization: You’ll sort dogs into batches based on their check-in times and days.
- Calculation: You’ll calculate costs for each dog’s stay, considering premium memberships and hours of stay.
- Debugging: You’ll have the opportunity to debug your code, ensuring it works as expected.
By Navya Sharma, and Srimathi Vadivel
Staircase Builder – ## course points
The purpose of this assignment is to familiarize you with using 2D arrays.
Refer to our Programming Assignments FAQ for instructions on how to install VScode, how to use the command line and how to submit your assignments.
Programming
Write a program and submit on Autolab. We provide this zip file containing Staircase.java, and _____ For this problem, update and submit the corresponding file.
Observe the following rules:
- DO NOT use System.exit()
- DO NOT add any import statements
- DO NOT add the project or package statements
- DO NOT change the class name
- DO NOT change the headers of ANY of the given methods
- DO NOT add any new class fields
- ONLY print the result as specified by the example for each problem.
- DO NOT print other messages, follow the examples for each problem.
Staircase.java (## points) Write a program, Staircase.java, that reads 2 integer arguments from the command line, the first argument being n, the size of an n * n square array, and the second argument being the total number of bricks to build a staircase. The program should print out a 2D array, marked with an “X” to indicate brick placement, and “ ” where bricks are not placed. This should be followed by a statement indicating the number of bricks remaining after building the staircase. Make sure bricks are placed from the bottom up. It is important to remember that your staircase must maintain an ascending order, where each step up, consists of one less brick placed than the previous step. Make sure that “Bricks remaining: ” is written correctly. Computers are not smart and will mark it as wrong, therefore, penalizing you.
Getting help
If anything is unclear, don’t hesitate to drop by office hours or post a question on Piazza. Find instructors’ office hours by clicking the Staff link from the course website. In addition to office hours we have the CAVE (Collaborative Academic Versatile Environment), a community space staffed with lab assistants which are undergraduate students further along the CS major to answer questions.
By Tanvi Yamarthy, and Vidushi Jindal
2022

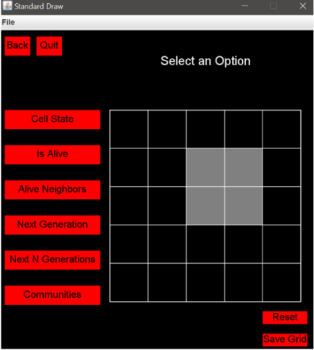

Conway Game of Life – 98 course points
The purpose of this assignment is to practice your understanding of 2D arrays and union-find.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
The assignment has two components:
- Coding (95 points) submitted through Autolab.
- Reflection (3 points) submitted through a form.
- Submit the reflection AFTER you have completed the coding component.
- Be sure to sign in with your RU credentials! (netid@scarletmail.rutgers.edu)
- You cannot resubmit reflections but you can edit your responses before the deadline by clicking the Google Form link, signing in with their netid, and selecting “Edit your response”
Overview
Conway Game of Life is a cellular discrete model of computation devised by John Horton Conway. The game consists of a game board (grid) of n x m cells, each in one of two states, alive or dead.
The game starts with an initial pattern, then it will change what cells are alive or dead from one generation to the next depending on a set of rules. As the Game of Life continues, the game will keep making a new generation (based on the preceding one) until it reaches one of three states.
By Max Goldberg and Seth Kelley
Merkle Trees – 100 course points
This assignment aims to simulate the functions and underlying processes of Github while using Merkle Trees.
GitHub:
GitHub is a code hosting platform for version control and collaboration. It lets you and others work together on projects from anywhere. Here we will go over the basic functionality and elements of Github so that the tasks in the assignment can be more easily understood.
Git is a tool you can download to have version control over your code. Version control refers to managing and keeping track of different and past versions of your code. The folder that contains all of the code you want to keep track of for your project is know as a repository, or repo for short. GitHub is an online, cloud-based service for managing your repositories. It allows users to not just create repositories where they can store their code, but also to add collaborators to build a project together. With these tools, users can go back to previous versions of their code, work on multiple different versions, or “branches”, of their code at once, users can work on different features of the code separately yet simultaneously, and much more. When someone wants to make changes to a GitHub repository, the person typically creates a new “branch” that is essentially like a copy of the original to work on something new. The original or the “master” branch is supposed to contain complete working code. For the purposes of this project, we will not be using branches but will simulate different users working from their local systems and collaborating.
While repositories are stored in the cloud on GitHub, users can use Git to locally manage them on their computer. Git is a command-line tool, meaning it is used by typing commands into the terminal, just like how using Java requires the commands java and javac. The main repository stored on GitHub is known as the master branch, repository, or version, while Git allowed users to locally work with a copy of the master repository. For this assignment, we do not own GitHub, so this master branch and any clones made will all be stored on your computer. The following commands provide the basic functionality of Git:
- git clone – copies, or “clones”, all of the files in a specified GitHub repository into a folder on the user’s own local machine
- git commit – saves, or “commits”, the current state of the repository, similar to saving your progress in a game or storing a snapshot of all your code
- git push – replaces the master repository with the state of your local repository that you last committed. This is known as “pushing” your code to the master repository, which results in the master now including all your changes to the code and makes it available to other collaborators
- git status – tells you the current status of your local repository with respect to the version stored on GitHub and provides information on which files were changed and the difference in commits. For the purposes of this assignment, we will use a simplified version of git status that only identifies if local code is different from the master branch.
Git and GitHub are able to deliver these valuable functionalities very quickly by using Merkle Trees.
Merkle Trees (also known as hash trees) are a type of binary tree in which each Node contains a hash value based upon its children nodes. They allow for efficient verification of content and are most notably used in Bitcoin to verify transactions. A hash value is a uniquely generated value based on the information that is being hashed. This means if the contents of two text files are different, the hash values of each file would be different.
The leaf nodes contain a hash of some sort of data or file and every other inner node contains a hash of the hashes of its children. For example, L1 through L4 are data blocks/files. A hash value is computed and stored in the leaf nodes 0-0, 0-1, 1-0, 1-1. Every other inner node contains a hash value computed from the child nodes. The inner Node Hash 0 contains a hash computed from Hash 0-0 and 0-1.
The usefulness of this tree is rooted in the fact that we can tell instantly if a data block/file was changed by comparing the root node hash values. Along with that we can identify which data blocks/files were changed by going down the tree and comparing hash values.
Your task in this assignment is to replicate the functions of GitHub through the use of Merkle trees
By Sana Shaikh and Harsh Samayamantula
Hunger Games – 100 course points
In this assignment, you will practice implementing binary search trees.
READ the assignment as soon as it comes out! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
Overview
The goal of this assignment is to simulate the Hunger Games. It is a modified version of the original Hunger Games.
The Hunger Games is a fictional annual event where one pair of people is chosen from each district by lottery and competes in a battle royale to the death. The goal of this assignment is to use Binary Search Trees to run your own version of the games through the input files of People and Districts provided to you in this assignment.
- People are placed into districts and put into odd and even populations within each district. Districts are defined by an ID value and all districts can be stored through a binary search tree using ID’s as key values for comparisons. Odd and even populations are based on whether each person’s birth month is odd or even. For example, January (01) birth months would be odd, while December (12) birth months would be even.
In other words, we can represent the Hunger Games districts as a binary search tree, assigning identifiers (district ID’s) to each district in order to make comparisons.
By Kal Pandit, Pranay Roni, and Maksims Kurjanovics Kravcenko
RU Kindergarten – 100 course points
The purpose of this assignment is to practice your understanding of linked list structures.
This assignment will take longer to complete than the first assignment.
Start your assignment early! You will need time to understand this assignment and the many questions it may present.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
Overview
In this assignment you will simulate activities in a kindergarten classroom.
You will simulate the students standing in a line, the students on their seats, and the students playing musical chairs.
The assignment uses:
- a Singly Linked List to simulate student standing in a line.
- a 2D array to simulate students at their seats.
- a Circular Linked List to simulate students sitting on chairs to play the musical chairs game.
By Kal Pandit, Ethan Chou, and Maksims Kurjanovics Kravcenko
RUBuses Write Up – 100 course points
The purpose of this assignment is to practice your understanding of directed graphs and the adjacency list graph storage.
This assignment will take a SUBSTANTIAL amount of time to complete and is SUBSTANTIALLY harder than the previous assignments.
Start your assignment early! You need time to understand the assignment and to answer the many questions that will arise as you read the description and the code provided.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
Overview
At Rutgers University-New Brunswick, we have a chain of bus routes that resemble a similar structure to what’s being learned in class: a graph. Directed to be specific. If we pay attention to the picture [attached], we can see that every bus stop has connections to others, especially those that have multiple bus routes stopping at a specific stop, creating a web-like structure. In this assignment, you will learn how to create a directed graph that can be applied to the real-world basics, in this case, of transportation.
Explanation: Each bus stop has connections to it’s neighboring bus stops represented using edges. Each edge has a distance and a speed, or speed limit, that you can go on the edges. The reason why the graph is directed is because each bus goes in one direction in the graph of bus stops. For example, the LX bus goes from Livi to College Ave and then back to Livi and then College Ave, etc . It creates a directed cycle.
Note: Each bus traverses through the graph, and multiple buses can traverse through the graph. The bus stops are at a given location, so essentially, the names of the bus stops are the bus stops at a given location. For example, a bus stop at Hill Center would be called Hill Center.
Made by Nikhil Munagala and Maksims Kurjanovics Kravcenko
RU Trick or Treating – 100 course points
Overview:
When going Trick-or-Treating, there are many neighborhoods, houses, candy baskets, and types of candy to go through. We can represent the network these neighborhoods, houses, baskets, and candies form as a weighted undirected graph – this way we can find out the ways children can get the most treats, the shortest routes children can take, whether children can complete a route fast enough, and measure other important factors.
Start your assignment early! This assignment will take a substantial amount of time to complete compared to previous assignments.
By Kal Pandit and Seth Kelley
Play 2048 – 100 course points
In this assignment, you will be coding the game 2048 using 2-D arrays. You will practice array manipulation, how to navigate references to objects, and the object-oriented programming (OOP) paradigm.
Be sure to start your assignment early – don’t wait until the last minute! You will need time to understand this assignment and the many questions it may present.
Refer to our Programming Assignments FAQ for instructions on how to install VSCode, how to use the command line and how to submit your assignments.
See this video on how to import the project into VSCode and how to submit into Autolab.
Overview
2048 is a puzzle game where you use the arrow keys to move left, right, up or down to merge tiles of the same number together. Check it out if you never played it before.
The goal of the game is to keep merging numbered (non-zero) tiles until you get one 2048 tile. If two tiles with the same number touch each other, they are merged together in the direction swiped into a new tile with twice the value. (ex: if you move up, the topmost value doubles and the bottom value becomes 0). Be careful with your moves – if the board is full at the end of a turn and there are no other valid moves, it’s game over!
In this assignment, we will represent the 2048 grid as an array of integers of arbitrary size (at least 2×2), with 0’s representing empty tiles. You can test individual methods using grids of arbitrary size, but when playing the full game in the Driver, the grid will be 4×4. Do not assume that all grids are exactly 4×4. Note that we will grade your individual operations – not your score or ability to get to 2048.
By Kal Pandit and Ishaan Ivaturi